RFID is an indispensable device in many people’s daily lives, such as building access control and elevator access control. Many companies also use RFID for attendance management. The most commonly used RFID module is RC522. We use the simplest program to read the UID of the card and compare it with the stored UID. Then use it with OLED to turn it into a practical system!
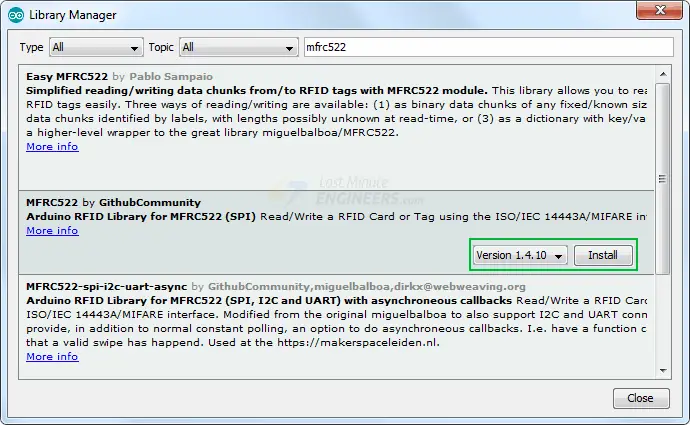
Before writing the program, connect all the wires first, because the OLED will be used later, so connect the OLED as well! If you don’t need OLED, don’t bother with the OLED part!
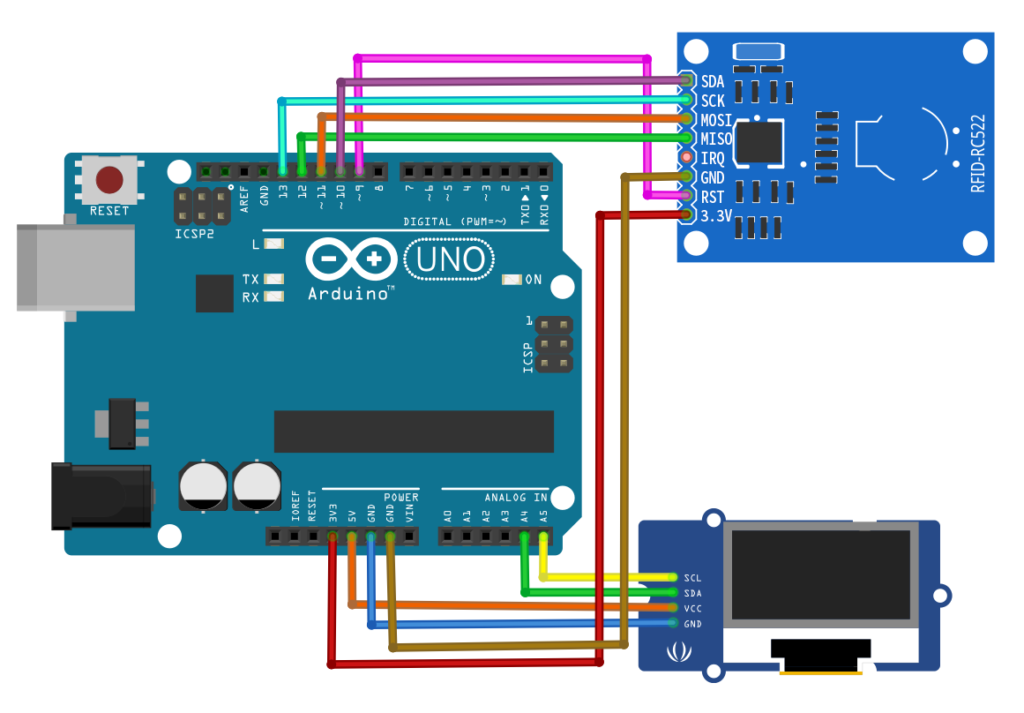
The next step is to install the function library. The most convenient and usable one that Jason has tested, the MFRC522 function library must be the first, and it can be downloaded directly from the program manager.
Let’s first write a program to read the UID of the RFID card or magnetic buckle. The UID is unique to each card and is not repeated. Therefore, most access control with low security levels directly identify each card from the UID. A card.
Program: Read the card UID and view it from the monitoring window
#include <SPI.h>
#include <MFRC522.h>
#define RST_PIN 9
#define SS_PIN 10 //This is the SDA pin on the module
MFRC522 mfrc522; // Create MFRC522 entity
void setup() {
Serial.begin(9600);
SPI.begin(); // Initialize SPI interface
mfrc522.PCD_Init(SS_PIN, RST_PIN); // Initialize MFRC522 card
Serial.print(F("Reader "));
Serial.print(F(": "));
mfrc522.PCD_DumpVersionToSerial(); // Display the version of the card reading device
}
void loop() {
// Check if it is a new card
if (mfrc522.PICC_IsNewCardPresent() && mfrc522.PICC_ReadCardSerial()) {
//Display card content
Serial.print(F("Card UID:"));
dump_byte_array(mfrc522.uid.uidByte, mfrc522.uid.size); // Display the UID of the card
Serial.println();
Serial.print(F("PICC type: "));
MFRC522::PICC_Type piccType = mfrc522.PICC_GetType(mfrc522.uid.sak);
Serial.println(mfrc522.PICC_GetTypeName(piccType)); //Display the card type
mfrc522.PICC_HaltA(); // Card enters stop mode
}
}
/**
* This subroutine displays the read UID in hexadecimal
*/
void dump_byte_array(byte *buffer, byte bufferSize) {
for (byte i = 0; i < bufferSize; i++) {
Serial.print(buffer[i] < 0x10 ? " 0" : " ");
Serial.print(buffer[i], HEX);
}
}
;
This program is adapted from the example attached to Jason’s simplified function library. The following line will detect the version of the RFID device. It basically detects whether the device is operating normally.
mfrc522.PCD_DumpVersionToSerial()
After reading the card, the most important UID is stored in mfrc522.uid.uidByte. Use the dump_byte_array subroutine to display the UID in the monitoring window.
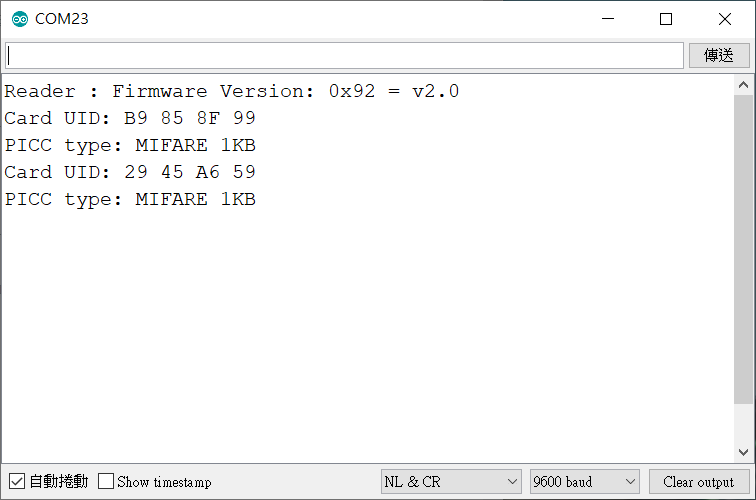
The program is not difficult and everyone should be able to complete it.
We have got the UID of the card, write it down, and then we have to write a program to determine whether the recorded card has been detected.
Program: Determine whether the card UID is passed, view it from the monitoring window
#include <SPI.h>
#include <Wire.h>
#include <MFRC522.h>
#define RST_PIN 9
#define SS_PIN 10 //SDA on RC522 card
MFRC522 mfrc522; // Create MFRC522 entity
char *reference;
byte uid[]={0x49, 0xE5, 0xA0, 0xC1}; //This is the card UID we specified. The UID of the specific card can be obtained by the program that reads the UID, and then modify this line
void setup()
{
Serial.begin(9600);
SPI.begin();
mfrc522.PCD_Init(SS_PIN, RST_PIN); // Initialize MFRC522 card
Serial.print(F("Reader "));
Serial.print(F(": "));
mfrc522.PCD_DumpVersionToSerial(); // Display the version of the card reading device
}
void loop() {
//Serial.print("reading...");
// Check if a new card is detected
if (mfrc522.PICC_IsNewCardPresent() && mfrc522.PICC_ReadCardSerial()) {
//Display the UID of the card
Serial.print(F("Card UID:"));
dump_byte_array(mfrc522.uid.uidByte, mfrc522.uid.size); // Display the UID of the card
Serial.println();
Serial.print(F("PICC type: "));
MFRC522::PICC_Type piccType = mfrc522.PICC_GetType(mfrc522.uid.sak);
Serial.println(mfrc522.PICC_GetTypeName(piccType)); //Display the card type
//Compare the obtained UID with the UID we specified
bool they_match = true; // The initial value is assumed to be true
for ( int i = 0; i < 4; i++ ) { // The card UID has 4 segments, and they are compared separately.
if ( uid[i] != mfrc522.uid.uidByte[i] ) {
they_match = false; // If any match is incorrect, they_match is false and the match ends.
break;
}
}
//Display the comparison results in the monitoring window
if(they_match){
Serial.print(F("Access Granted!"));
}else{
Serial.print(F("Access Denied!"));
}
mfrc522.PICC_HaltA(); // Card enters stop mode
}
}
/**
* This subroutine displays the read UID in hexadecimal
*/
void dump_byte_array(byte *buffer, byte bufferSize) {
for (byte i = 0; i < bufferSize; i++) {
Serial.print(buffer[i] < 0x10 ? " 0" : " ");
Serial.print(buffer[i], HEX);
}
}
The program is roughly the same as the previous one for reading UID. The bottom line is the most important. Please replace it with the UID of one of the cards you read before so that we can compare it.
byte uid[]={0x49, 0xE5, 0xA0, 0xC1};
The next step is to use the bottom two pieces of code to determine whether the inserted card is exactly the same as the UID we recorded.
//Compare the obtained UID with the UID we specified
bool they_match = true; // The initial value is assumed to be true
for ( int i = 0; i < 4; i++ ) { // The card UID has 4 segments, and they are compared separately.
if ( uid[i] != mfrc522.uid.uidByte[i] ) {
they_match = false; // If any match is incorrect, they_match is false and the match ends.
break;
}
}
//Display the comparison results in the monitoring window
if(they_match){
Serial.print(F("Access Granted!"));
}else{
Serial.print(F("Access Denied!"));
}
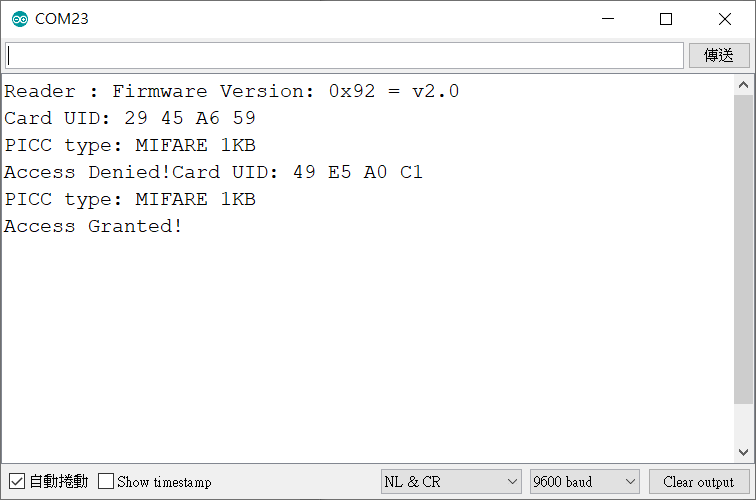
Next, we attach two programs that combine OLED. This time Jason uses the u8g2 library to work with 0.96-inch OLED. For the OLED part, please refer to Jason’s previous article.
Program: Read card UID and view from OLED
#include <Arduino.h>
#include <U8g2lib.h>
#include <SPI.h>
#include <Wire.h>
#include <MFRC522.h>
//Two pins used by MFRC522
#define RST_PIN 9
#define SS_PIN 10 //SDA on RC522 card
MFRC522 mfrc522; // Create MFRC522 entity
char *reference; //Text showing whether the device is normal or not
//This line is used by NodeOLED
//U8G2_SSD1306_128X64_NONAME_1_SW_I2C u8g2(U8G2_R0, /* clock=*/ D2, /* data=*/ D1, /* reset=*/ U8X8_PIN_NONE);
U8G2_SSD1306_128X64_NONAME_1_HW_I2C u8g2(U8G2_R0, /* reset=*/ U8X8_PIN_NONE); //Use this line for Arduino Uno+0.96-inch OLED
//U8G2_SH1106_128X64_NONAME_1_HW_I2C u8g2(U8G2_R0, /* reset=*/ U8X8_PIN_NONE); //If you use 1.3-inch OLED, use this line
//The title above the OLED is represented graphically
static const unsigned char PROGMEM title[256] = {
0XFF,0XFF,0XFF,0XFF,0XFF,0XFF,0XFF,0XFF,0XBF,0XEF,0XFE,0XFF,0XFF,0XFF,0XFF,0XFF,
0XFF,0XFF,0XFF,0XFF,0XFF,0XFF,0XFD,0XE7,0XBF,0XEF,0XFA,0XFF,0XFF,0XFF,0XFD,0XFE,
0XE3,0X3F,0XF8,0XC1,0X1F,0XFE,0XFB,0XEE,0X7F,0X83,0XFA,0XFF,0XE3,0XFF,0X7E,0XFE,
0XDB,0XBF,0XFF,0XF7,0XDF,0XFD,0XE0,0X82,0XFF,0XFF,0XF6,0X1F,0XFC,0XFF,0X12,0XE0,
0XBB,0XBF,0XFF,0XF7,0XDF,0XFB,0XFF,0XFE,0X0F,0XB6,0XFE,0XE1,0XFE,0X7F,0XBB,0XFF,
0XBB,0XBF,0XFF,0XF7,0XDF,0XF7,0XA5,0XB6,0XFF,0X03,0XF0,0X3F,0XFF,0XBF,0XBB,0XFB,
0XBB,0XBF,0XFF,0XF7,0XDF,0XF7,0XB5,0XD6,0X1F,0XFE,0XFE,0XDF,0XF3,0X1F,0XDC,0XFB,
0XBB,0XBF,0XFF,0XF7,0XDF,0XF7,0XB7,0XDE,0XFF,0X83,0XF6,0X07,0XFC,0XFF,0XDE,0XF3,
0XDB,0X3F,0XF8,0XF7,0XDF,0XF7,0XA0,0X82,0X1F,0XBA,0XFA,0X7F,0XE6,0X7F,0X0B,0XF4,
0XE3,0XBF,0XFF,0XF7,0XDF,0XF7,0XFB,0XEE,0XFF,0XBB,0XFA,0X9F,0XDF,0XBF,0XB9,0XE5,
0XDB,0XBF,0XFF,0XF7,0XDF,0XF7,0XFB,0XEE,0X1F,0X82,0XFD,0X03,0X80,0X1F,0XBE,0XFD,
0XDB,0XBF,0XFF,0XF7,0XDF,0XF7,0XE0,0X82,0XDF,0XBA,0XFD,0XFB,0XBE,0XFF,0XB3,0XFD,
0XDB,0XBF,0XFF,0XF7,0XDF,0XFB,0XFB,0XEE,0XDF,0XBA,0XFE,0XCF,0XF6,0XBF,0XB6,0XFD,
0XBB,0XBF,0XFF,0XF7,0XDF,0XFD,0X7B,0XEF,0XDF,0X82,0XF4,0XE7,0XCE,0XBF,0XD5,0XED,
0XBB,0XBF,0XFF,0XC1,0X1F,0XFE,0X7D,0XEF,0X1F,0X7A,0XF5,0XF9,0XBE,0XBF,0XDD,0XED,
0XFF,0XFF,0XFF,0XFF,0XFF,0XFF,0XBD,0XEF,0XDF,0XBF,0XFB,0X1F,0XFE,0XDF,0XE7,0XF3,
};
void setup()
{
Serial.begin(9600);
SPI.begin(); // Initialize SPI interface
u8g2.begin();
mfrc522.PCD_Init(SS_PIN, RST_PIN); // Initialize MFRC522 card
Serial.print(F("Reader "));
Serial.print(F(": "));
mfrc522.PCD_DumpVersionToSerial(); // Display the version of the card reading device
//Test whether the RFID device is normal
byte v = mfrc522.PCD_ReadRegister(mfrc522.VersionReg);
if((v == 0x00) || (v == 0xFF)){
reference = "Reader Error";
showText1();
}else{
reference = "Reader Ready";
showText1();
delay(1500);
showText2();
}
}
//Display the first line of text
void showText1(){
u8g2.setFont(u8g2_font_samim_16_t_all); //Font
u8g2.firstPage();
do {
u8g2.drawXBMP(0,0, 128, 16, title);
u8g2.drawStr(0,40,"Reader Testing...");
u8g2.drawStr(10,60,reference);
} while ( u8g2.nextPage() );
}
//Display the second line of text
void showText2(){
char *reference;
u8g2.setFont(u8g2_font_samim_16_t_all); //Font
u8g2.firstPage();
do {
u8g2.drawXBMP(0,0, 128, 16, title);
u8g2.drawStr(10,40,"Place RFID...");
} while ( u8g2.nextPage() );
}
void loop() {
//Serial.print("reading...");
// Check if a new card is detected
if (mfrc522.PICC_IsNewCardPresent() && mfrc522.PICC_ReadCardSerial()) {
//Display card content
Serial.print(F("Card UID:"));
dump_byte_array(mfrc522.uid.uidByte, mfrc522.uid.size); // Display the UID of the card to the monitoring window
//Display the card UID; the content of mfrc522.uid.uidByte is the UID, and the data is an array of bytes. We need to take them out one by one and display them on the OLED.
for(int i = 0; i < mfrc522.uid.size; i++)
{
u8g2.firstPage();
do {
u8g2.drawXBMP(0,0, 128, 16, title);
u8g2.setCursor(10,40);
u8g2.print("RFID UID:");
for (byte i = 0; i < mfrc522.uid.size; i++) {
if(mfrc522.uid.uidByte[i] < 0x10){ //When reading a number less than 10, an extra zero will be displayed in front
u8g2.setCursor(10+i*25,60);
u8g2.print("0");
u8g2.setCursor(10+i*25+10,60);
u8g2.print(mfrc522.uid.uidByte[i], HEX); //Use HEX to display a UID, such as A0 or C1, etc.
}else{
u8g2.setCursor(10+i*25,60);
u8g2.print(mfrc522.uid.uidByte[i], HEX);
}
}
} while ( u8g2.nextPage() );
}
Serial.println();
Serial.print(F("PICC type: "));
MFRC522::PICC_Type piccType = mfrc522.PICC_GetType(mfrc522.uid.sak);
Serial.println(mfrc522.PICC_GetTypeName(piccType)); //Display the card type
mfrc522.PICC_HaltA(); // Card enters stop mode
}
}
/**
* This subroutine displays the read UID in hexadecimal
*/
void dump_byte_array(byte *buffer, byte bufferSize) {
for (byte i = 0; i < bufferSize; i++) {
Serial.print(buffer[i] < 0x10 ? " 0" : " ");
Serial.print(buffer[i], HEX);
}
}
Program: Determine whether the card UID is passed and check it on the OLED
#include <Arduino.h>
#include <U8g2lib.h>
#include <SPI.h>
#include <Wire.h>
#include <MFRC522.h>
#define RST_PIN 9
#define SS_PIN 10 //SDA on RC522 card
MFRC522 mfrc522; // Create MFRC522 entity
char *reference;
byte uid[]={0x49, 0xE5, 0xA0, 0xC1}; //This is the card UID we specified. The UID of the specific card can be obtained by the program that reads the UID, and then modify this line
//This line is used by NodeOLED
//U8G2_SSD1306_128X64_NONAME_1_SW_I2C u8g2(U8G2_R0, /* clock=*/ D2, /* data=*/ D1, /* reset=*/ U8X8_PIN_NONE);
U8G2_SSD1306_128X64_NONAME_1_HW_I2C u8g2(U8G2_R0, /* reset=*/ U8X8_PIN_NONE); //Use this line for Arduino Uno+0.96-inch OLED
//U8G2_SH1106_128X64_NONAME_1_HW_I2C u8g2(U8G2_R0, /* reset=*/ U8X8_PIN_NONE); //If you use 1.3-inch OLED, use this line
//The title above the OLED is represented graphically
static const unsigned char PROGMEM title[256] = {
0XFF,0XFF,0XFF,0XFF,0XFF,0XFF,0XFF,0XFF,0XBF,0XEF,0XFE,0XFF,0XFF,0XFF,0XFF,0XFF,
0XFF,0XFF,0XFF,0XFF,0XFF,0XFF,0XFD,0XE7,0XBF,0XEF,0XFA,0XFF,0XFF,0XFF,0XFD,0XFE,
0XE3,0X3F,0XF8,0XC1,0X1F,0XFE,0XFB,0XEE,0X7F,0X83,0XFA,0XFF,0XE3,0XFF,0X7E,0XFE,
0XDB,0XBF,0XFF,0XF7,0XDF,0XFD,0XE0,0X82,0XFF,0XFF,0XF6,0X1F,0XFC,0XFF,0X12,0XE0,
0XBB,0XBF,0XFF,0XF7,0XDF,0XFB,0XFF,0XFE,0X0F,0XB6,0XFE,0XE1,0XFE,0X7F,0XBB,0XFF,
0XBB,0XBF,0XFF,0XF7,0XDF,0XF7,0XA5,0XB6,0XFF,0X03,0XF0,0X3F,0XFF,0XBF,0XBB,0XFB,
0XBB,0XBF,0XFF,0XF7,0XDF,0XF7,0XB5,0XD6,0X1F,0XFE,0XFE,0XDF,0XF3,0X1F,0XDC,0XFB,
0XBB,0XBF,0XFF,0XF7,0XDF,0XF7,0XB7,0XDE,0XFF,0X83,0XF6,0X07,0XFC,0XFF,0XDE,0XF3,
0XDB,0X3F,0XF8,0XF7,0XDF,0XF7,0XA0,0X82,0X1F,0XBA,0XFA,0X7F,0XE6,0X7F,0X0B,0XF4,
0XE3,0XBF,0XFF,0XF7,0XDF,0XF7,0XFB,0XEE,0XFF,0XBB,0XFA,0X9F,0XDF,0XBF,0XB9,0XE5,
0XDB,0XBF,0XFF,0XF7,0XDF,0XF7,0XFB,0XEE,0X1F,0X82,0XFD,0X03,0X80,0X1F,0XBE,0XFD,
0XDB,0XBF,0XFF,0XF7,0XDF,0XF7,0XE0,0X82,0XDF,0XBA,0XFD,0XFB,0XBE,0XFF,0XB3,0XFD,
0XDB,0XBF,0XFF,0XF7,0XDF,0XFB,0XFB,0XEE,0XDF,0XBA,0XFE,0XCF,0XF6,0XBF,0XB6,0XFD,
0XBB,0XBF,0XFF,0XF7,0XDF,0XFD,0X7B,0XEF,0XDF,0X82,0XF4,0XE7,0XCE,0XBF,0XD5,0XED,
0XBB,0XBF,0XFF,0XC1,0X1F,0XFE,0X7D,0XEF,0X1F,0X7A,0XF5,0XF9,0XBE,0XBF,0XDD,0XED,
0XFF,0XFF,0XFF,0XFF,0XFF,0XFF,0XBD,0XEF,0XDF,0XBF,0XFB,0X1F,0XFE,0XDF,0XE7,0XF3,
};
void setup()
{
Serial.begin(9600);
SPI.begin(); // Initialize SPI interface
u8g2.begin();
mfrc522.PCD_Init(SS_PIN, RST_PIN); // Initialize MFRC522 card
Serial.print(F("Reader "));
Serial.print(F(": "));
mfrc522.PCD_DumpVersionToSerial(); // Display the version of the card reading device
//Test whether the RFID device is normal
byte v = mfrc522.PCD_ReadRegister(mfrc522.VersionReg);
if((v == 0x00) || (v == 0xFF)){
reference = "Reader Error";
showText1();
}else{
reference = "Reader Ready";
showText1();
delay(1500);
showText2();
}
}
void showText1(){
u8g2.setFont(u8g2_font_samim_16_t_all); //Font
u8g2.firstPage();
do {
u8g2.drawXBMP(0,0, 128, 16, title);
u8g2.drawStr(0,40,"Reader Testing...");
u8g2.drawStr(10,60,reference);
} while ( u8g2.nextPage() );
}
void showText2(){
char *reference;
u8g2.setFont(u8g2_font_samim_16_t_all); //Font
u8g2.firstPage();
do {
u8g2.drawXBMP(0,0, 128, 16, title);
u8g2.drawStr(10,40,"Place RFID...");
} while ( u8g2.nextPage() );
}
void loop() {
//Serial.print("reading...");
// Check if a new card is detected
if (mfrc522.PICC_IsNewCardPresent() && mfrc522.PICC_ReadCardSerial()) {
//Display the UID of the card
Serial.print(F("Card UID:"));
dump_byte_array(mfrc522.uid.uidByte, mfrc522.uid.size); // Display the UID of the card
for(int i = 0; i < mfrc522.uid.size; i++)
{
u8g2.firstPage();
do {
u8g2.drawXBMP(0,0, 128, 16, title);
u8g2.setCursor(10,40);
u8g2.print("RFID UID:");
for (byte i = 0; i < mfrc522.uid.size; i++) {
if(mfrc522.uid.uidByte[i] < 0x10){
u8g2.setCursor(10+i*25,60);
u8g2.print("0");
u8g2.setCursor(10+i*25+10,60);
u8g2.print(mfrc522.uid.uidByte[i], HEX);
}else{
u8g2.setCursor(10+i*25,60);
u8g2.print(mfrc522.uid.uidByte[i], HEX);
}
}
} while ( u8g2.nextPage() );
}
Serial.println();
Serial.print(F("PICC type: "));
MFRC522::PICC_Type piccType = mfrc522.PICC_GetType(mfrc522.uid.sak);
Serial.println(mfrc522.PICC_GetTypeName(piccType)); //Display the card type
//Compare the obtained UID with the UID we specified
bool they_match = true; // The initial value is assumed to be true
for ( int i = 0; i < 4; i++ ) { // The card UID has 4 segments, and they are compared separately.
if ( uid[i] != mfrc522.uid.uidByte[i] ) {
they_match = false; // If any match is incorrect, they_match is false and the match ends.
break;
}
}
Serial.println(they_match);
//Display the comparison results on OLED
u8g2.firstPage();
do {
u8g2.drawXBMP(0,0, 128, 16, title);
if(they_match){
u8g2.drawStr(10,40,"Access Granted");
}else{
u8g2.drawStr(10,40,"Access Denied");
}
} while ( u8g2.nextPage() );
delay(1500);
u8g2.firstPage();
do {
u8g2.drawXBMP(0,0, 128, 16, title);
u8g2.drawStr(10,40,"Place RFID...");