Arduino itself does not have a clock function, so it must rely on other modules to provide the time function. The most common one is the DS1302 time module. The method of use is quite simple. As long as you find the right function library, you can easily write a program.
Please install the Rtc by Makuna time module library first, which can be found in the program manager of Arduino IDE.
Wiring is not difficult, DS1302 has 3 signal lines, which can be modified from the program. 1602 is the I2C interface, SDA is connected to A4, and SCL is connected to A5. Everyone should be familiar with it.
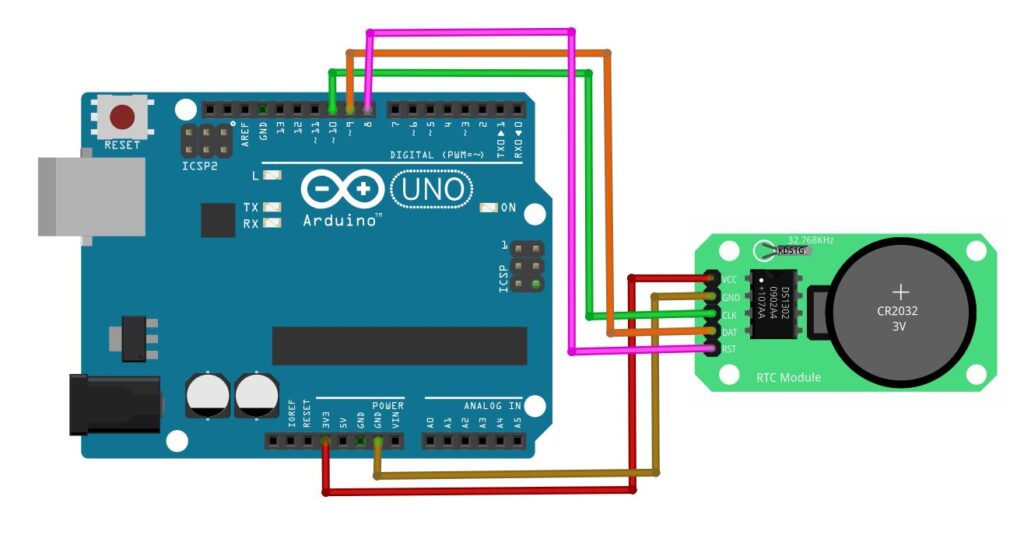
There are many function libraries for DS1302. Jason prefers to use the Rtc by Makuna time module library. The time setting method used in the examples provided is fully automatic, and the compiled time is automatically obtained for comparison with DS1302. , DS1302 will be updated if the time is slow. We do not need to manually key it, which is very convenient for beginners.
This example is very simple. After we get the date and time, we display it in the monitoring window.
#include <Wire.h>
#include <ThreeWire.h>
#include <RtcDS1302.h>
// DS1302 Wiring instructions: can be modified according to needs
// DS1302 CLK/SCLK --> 10
// DS1302 DAT/IO --> 9
// DS1302 RST/CE --> 8
// DS1302 VCC --> 3.3v - 5v
// DS1302 GND --> GND
ThreeWire myWire(9, 10, 8); // IO, SCLK, CE
RtcDS1302<ThreeWire> Rtc(myWire);
void setup ()
{
Serial.begin(9600);
Serial.print("compiled: ");
Serial.print(__DATE__);
Serial.println(__TIME__);
Rtc.Begin();
//__DATE__,__TIME__,is the date and time when the code was compiled
RtcDateTime compiled = RtcDateTime(__DATE__, __TIME__);
printDateTime(compiled);
Serial.println();
//判斷DS1302Is it connected?
if (!Rtc.IsDateTimeValid())
{
// Common Causes:
// 1) first time you ran and the device wasn't running yet
// 2) the battery on the device is low or even missing
Serial.println("RTC lost confidence in the DateTime!");
Rtc.SetDateTime(compiled);
}
if (Rtc.GetIsWriteProtected())
{
Serial.println("RTC was write protected, enabling writing now");
Rtc.SetIsWriteProtected(false);
}
if (!Rtc.GetIsRunning())
{
Serial.println("RTC was not actively running, starting now");
Rtc.SetIsRunning(true);
}
//Determine the time when DS1302 was first green and the time when it was compiled, which one is newer?
//If the compilation time is relatively new, set it up and change the time on DS1302 to the new time.
//now:The time of DS1302 last year, compiled: the time of compilation
RtcDateTime now = Rtc.GetDateTime();
if (now < compiled)
{
Serial.println("RTC is older than compile time! (Updating DateTime)");
//The compilation time is relatively new. Change the time on DS1302 to the compilation time.
Rtc.SetDateTime(compiled);
}
else if (now > compiled)
{
Serial.println("RTC is newer than compile time. (this is expected)");
}
else if (now == compiled)
{
Serial.println("RTC is the same as compile time! (not expected but all is fine)");
}
}
void loop ()
{
RtcDateTime now = Rtc.GetDateTime();
printDateTime(now);
Serial.println();
//Determine whether the DS1302 is normal. If not, it is usually because the wires are not connected properly or the battery is dead.
if (!now.IsValid())
{
Serial.println("RTC lost confidence in the DateTime!");
}
delay(10000); // Update every 10 seconds
}
#define countof(a) (sizeof(a) / sizeof(a[0]))
//Subprogram to display complete year, month, day and time
void printDateTime(const RtcDateTime& dt)
{
char datestring[20];
snprintf_P(datestring,
countof(datestring),
PSTR("%02u/%02u/%04u %02u:%02u:%02u"),
dt.Month(),
dt.Day(),
dt.Year(),
dt.Hour(),
dt.Minute(),
dt.Second() );
Serial.print(datestring);
}
